Snake Game Python Code
In this tutorial you will learn how to build the game snake. The game is an arcade game and it has very simple logic, which is why it is an ideal example to demonstrate how to build games with Pygame. The player is represented as snake, which grows if it eats an apple. Project: Snake Game in Python with source code. About Project. This simple Snake Game project is written in Python. The project file contains image files and python scripts (game.py, duplicate.py). GUI uses pygame library. Talking about the gameplay, it’s a single player game, where the player (Snake) has to eat all the apples in order to. I had an issue at line 29, within the 'while key!= 27' loop. Using python 3 somethingsomething - whatever the latest is at the time of posting - I was told I was getting a float, when it was expecting an integer. I wrote a simple Python snake game which is about 250 lines of code. Can someone give me some advice on how I can refactor/make it better? Game.py # game.py - 3/22/2013 import pygame, sys, os from.
Snake game has been the best entertaining mobile game since 1990’s. Mostly in snake game, we need one snake and a food to eat. The snake goes to eat the food while the size of the snake increases slowly. The game will end when the snake bites himself after failing to eat the food. The game is simple and can’t be cheated. I am writing code for a simple snake game where the player controls the head of the snake (a box I created using graphics functions) to eat 'apples' (another box). I figured out how to get the box to move using the checkKey method in a while loop. I also figured out how to detect collisions between the snake.
I wrote a simple Python snake game which is about 250 lines of code. Can someone give me some advice on how I can refactor/make it better?
game.py
classes.py
PimgdSnake Game Python Worm
Ryan1 Answer
$begingroup$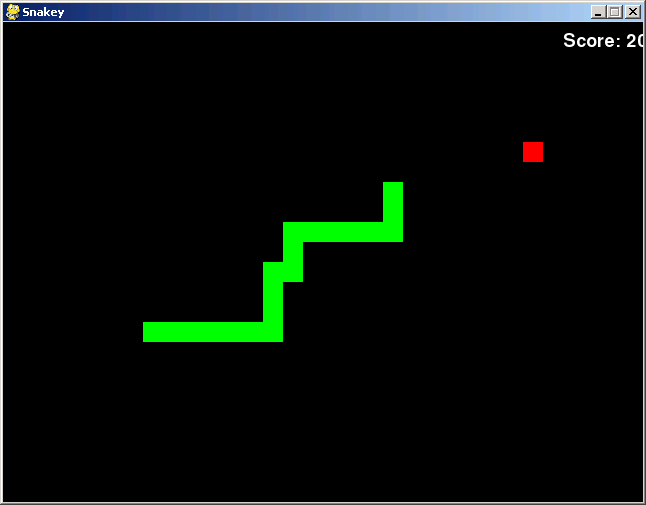
1. Introduction
This is not bad overall, considering that this is your first program written with PyGame. I've made many comments below, but don't take the length of this answer to heart: there are always many things to say about a piece of code of this length.
2. Game design issues
The game could do with some instructions. I had to look at the source code to see that I need to use WASD for movement. Alternatively, you might allow the player to use the arrow keys too (these are natural keys the player might try).
You use FPS (the number of frames per second) to control the speed of the snake. This design decision commits you to processing everything in the game at the same frequency as the snake moves. The concepts frames per second and speed of the snake in moves per second are distinct, so it's good practice to separate them.
At the moment there's nothing in the game other than the snake, so you get away with this. But as soon as you add other game elements that need to animate at different speeds, you'll run up against this difficulty. Better to get this right while things are still simple.
See section 5 for one way to solve this problem.
New pieces of food can be created in positions occupied by the snake!
The food position is not reset when a new game starts. (This can cause the snake to be overlap the food at the start of the game.)
The score doesn't get drawn during the game. Modio 3.0 download zip.
3. Major comments
The docstring for
collidesWithSelf
reads like this:This is not appropriate content for a docstring. The purpose of a docstring is to explain the interface of a method to a programmer who is trying to use it. But here you have some notes to yourself about the history of this function and why it is implemented like it is. These notes properly belong in a comment.
The reason you have been having problems in this function is that the growth of the snake is not right. In the
grow()
method you grow a new tail segment in the opposite direction to the snake's current movement. But this can cause the snake to self-intersect.The usual way that 'snake' games work is that when the snake eats some food, it does not grow a new tail segment immediately. Instead, it waits until the next time it moves and grows a new tail segment in the position where its old tail used to be. This is easily implemented by incrementing a counter each time the snake eats food:
and then decrementing the counter instead of deleting the tail segment:
This avoids self-intersection, and so this would allow you to implement the collision operation using your original approach. But you might consider this simpler approach:
You represent directions by numbers between 1 and 4. It is hard to remember which direction is which, so it would be easy to make a mistake and treat 1 as 'up' in one part of the code but 'down' in another. You'd be much less likely to make this mistake if you used the names
DIRECTION_UP
and so on. You went to all the trouble to create these names: why not use them?(But see 3.4 below for a better suggestion.)
The code below looks dodgy because there is no
else:
on the end of the series of tests.A programmer reading this would want to know what happens if
self.direction
is not in the range 1 to 4. Of course, you hope that you have designed the program so that this can't happen. So you might make this crystal clear by rewriting this code like this:(But see 3.4 below for a better suggestion.)
Instead of representing a direction with a number from 1 to 4 (which it's hard to remember which is which), why not use a pair (δx, δy)? For example, you could write:
This would save you a bunch of
if .. elif ..
tests. For example you could rewrite the code I gave above like this:Similarly, instead of having a bunch of
if . elif ..
tests for the input:you could have a lookup table that maps key to direction:
and then the test becomes:
You check for collision with the edges of the playing area like this:
but PyGame defines a
Rect
class with acollidepoint
method, so you could set up the rectangle like this inSnakeGame.__init__
:and then test for collision like this:
You number your coordinates starting at 1. This causes you some difficulty. For example, you have to subtract 1 from each of your coordinates before passing them to
pygame.draw.rect
. If your coordinates started at 0 you wouldn't have to do this.A lot of your code deals with positions or vectors represented by a pair (x, y). This means that each time you process a position, you have to break it down into its x and y coordinates, process the coordinates, and then reassemble the results into a new pair. For example:
You could simplify this code by making a class to represent positions and vectors. Sadly, PyGame does not come with such a class, but you can easily find many implementations on the web, or just write one yourself:
now you can write:
and make many other simplifications. (See section 5 for more methods on the
Vector
class.)
4. Minor comments
The Python style guide (PEP8) says that method names should 'Use the function naming rules: lowercase with words separated by underscores as necessary to improve readability'. So you should consider renaming
collidesWithSelf
ascollides_with_self
and so on. (You're not obliged to follow PEP8 but it makes it easier for other Python programmers to read your code.)The organization of the code into the files
game.py
andclasses.py
doesn't seem to be motivated by any principle, and the vague nameclasses.py
confirms this. For a small game like this, I don't think there's anything to be lost by putting all the code in one file. (And if it grows to the point where you want to split it, the obvious thing to do would be to put theSnake
class in its own module.)It's not clear to me what you gain from separating your game initialization code into
main
andSnakeGame.__init__
. Why not put all the initialization into the latter?You don't need
sys.exit()
at the end ofmain
: Python quits automatically when it finishes running your program. Adding this line just makes it hard to test your program from the interactive interpreter, because when you quit from the game, it exits the interactive interpreter too.You have unnecessary parentheses in many places. A line like:
can be written:
since comma binds more tighly than assignment in Python. 'Program as if you know the language'!
DIRECTON_DOWN
is misspelled. (Is this why you don't use it?)You represent the snake using a queue of positions, which is a good approach. However, you implement your queue using a Python list. The trouble here is that Python lists are efficient at adding and removing elements at the end, but not at the beginning. In particular the operation
takes time proportional to the length of
self.pieces
. (You can consult the TimeComplexity page on the Python wiki to see the time complexity of operations on built-in Python data structures.) This isn't a big deal here, since the snake never gets very long, but it's worth getting into practice at thinking about the complexity of your algorithms.For an efficient queue implementation, use
collections.deque
.The line:
can be rewritten:
Since negative list indexes count backwards from the end of the list.
Instead of doing division and coercing the result to an integer:
use Python's floor division operation:
Snake Game Code Python 3
5. Revised code
This code addresses the comments above and includes some more improvements for you to discover.
Gareth ReesGareth Reesprotected by Jamal♦Dec 17 '14 at 17:21
Thank you for your interest in this question. Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?